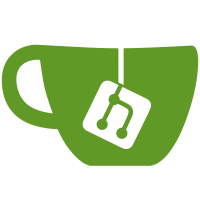
All checks were successful
Publish / build-and-push (push) Successful in 6m14s
Reasonably hacky, in that I introduce a facade to reuse the data format previously provided by the `csv` module, rather than using the `list[list[str]]` directly. Next I want to introduce something like Celery to continually refresh. Note that this will require changes to the deployment repo in order to provide the required secrets.
64 lines
2.2 KiB
Python
64 lines
2.2 KiB
Python
from google.oauth2 import service_account
|
|
from googleapiclient.discovery import build
|
|
import os
|
|
|
|
|
|
def get_data() -> list[list[str]]:
|
|
spreadsheet_id = os.getenv("SPREADSHEET_ID")
|
|
path_to_credentials = os.getenv("PATH_TO_GOOGLE_SHEETS_CREDENTIALS")
|
|
resp = []
|
|
# Hardcoding the format (and page names) to the spreadsheet that my group uses, because I'm lazy -
|
|
# should be easy to change those tousing environment variables if needed
|
|
for idx, year in enumerate(["2024", "2025"]):
|
|
# Assumes that the headers are the same for all years
|
|
if idx == 0:
|
|
resp = read_sheet(spreadsheet_id, f"{year} games", path_to_credentials)
|
|
else:
|
|
# Drop the headers from this year, because they were already added by the previous year.
|
|
resp.extend(
|
|
read_sheet(spreadsheet_id, f"{year} games", path_to_credentials)[1:]
|
|
)
|
|
return resp
|
|
|
|
|
|
# The scope for Google Sheets API
|
|
SCOPES = ["https://www.googleapis.com/auth/spreadsheets.readonly"]
|
|
|
|
|
|
def get_service_account_credentials(credentials_path):
|
|
"""Gets service account credentials from a JSON key file.
|
|
|
|
Args:
|
|
credentials_path: Path to the service account JSON key file
|
|
|
|
Returns:
|
|
Credentials object for the service account
|
|
"""
|
|
return service_account.Credentials.from_service_account_file(
|
|
credentials_path, scopes=SCOPES
|
|
)
|
|
|
|
|
|
def read_sheet(spreadsheet_id, range_name, credentials_path):
|
|
"""Reads data from a Google Sheet using service account credentials.
|
|
|
|
Args:
|
|
spreadsheet_id: The ID of the spreadsheet to read from
|
|
range_name: The A1 notation of the range to read from (e.g., 'Sheet1!A1:D10')
|
|
credentials_path: Path to the service account JSON key file
|
|
|
|
Returns:
|
|
A list of lists containing the values from the specified range
|
|
"""
|
|
creds = get_service_account_credentials(credentials_path)
|
|
service = build("sheets", "v4", credentials=creds)
|
|
|
|
# Call the Sheets API
|
|
sheet = service.spreadsheets()
|
|
result = (
|
|
sheet.values().get(spreadsheetId=spreadsheet_id, range=range_name).execute()
|
|
)
|
|
|
|
values = result.get("values", [])
|
|
return values
|